The US Military Recruit Training Programs are known for rapidly transforming individuals into servicemembers who are physically and mentally fit for their duties. They are exceedingly persistent and relentless when it comes to pushing recruits to new levels of maturity and performance. Giving any less than 100% is not an option.
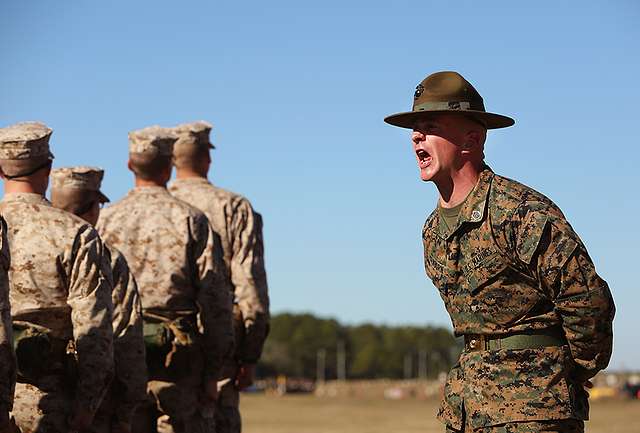
https://nara.getarchive.net/media/hurst-texas-native-a-marine-corps-drill-instructor-on-parris-island-e176b2
ChatGPT is not an intelligent being, but it’s enjoyable to treat it as such since it does quite well using a conversational style. It can also be lazy (theories on why later). The point of this post is to demonstrate how it’s possible to push ChatGPT beyond it’s early, sometimes meager efforts. This can be a valuable prompt tactic in maximizing your productivity with this tool.
NOTE: ChatGPT-4 was used for this post. Future posts might address whether GPT-3 might be sufficient for work like this.
Since Aviation is a passion of mine, I decided to have a session with ChatGPT to get some help decoding the Terminal Aerodrome Forecast (TAF) reports. These reports help pilots understand the weather for flight planning. They are heavily coded because they go way back to a time when transmitting the data was expensive. While it’s required for pilots to be able to read the coded messages, it’s of great help when they are decoded to plain English.
Another useful tactic is to not assume that ChatGPT can’t get ahold of the specification on it’s own. I start by testing for awareness for the win.
Are you aware of the Terminal Aerodrome Forecast and it’s related symbols?
Yes, I can certainly help with understanding Terminal Aerodrome Forecasts (TAFs) and their related symbols.
TAFs are weather forecast reports provided for aviation purposes. They are normally issued four times a day, every six hours, and typically have a forecast period of 24 hours (some major airports may have a forecast period of 30 hours).
They use a concise coding system which represents different weather conditions. Here are some common symbols and their meanings:
-
Wind – It’s denoted by the direction (in tens of degrees from true north) and speed (in knots). For example, "27015G25KT" means a wind coming from 270 degrees at 15 knots, gusting up to 25 knots.
-
Visibility – Given in statute miles or meters. For example, "9999" means a visibility of 10 kilometers or more.
-
Weather Phenomena – These are denoted by a combination of different letters:
- TS – Thunderstorm
- SH – Showers
- RA – Rain
- SN – Snow
- DZ – Drizzle
- FG – Fog
- BR – Mist
- HZ – Haze
- FU – Smoke
- VA – Volcanic ash
- DU – Widespread dust
- SA – Sand
- FZ – Freezing
-
Cloud Cover – This is described with a combination of cloud type and altitude (in hundreds of feet). For example, "BKN020" means ‘broken’ clouds at 2000 feet.
- FEW – Few clouds
- SCT – Scattered clouds
- BKN – Broken clouds
- OVC – Overcast clouds
-
Changes in Forecast – These are denoted by words like "BECMG" (becoming) or "TEMPO" (temporary).
-
Cavok – It means Ceiling and Visibility OK, implying no significant weather to report and visibility is greater than 10 km, and no cloud below 5000 feet or the highest minimum sector altitude.
These are just a few examples. A comprehensive list can be obtained from aviation regulatory bodies or aviation weather services like the FAA or the UK’s Met Office.
In reading these forecasts, one must understand that the terminology and coding system is designed for brevity and worldwide use, thus it’s a bit cryptic if you’re not familiar with it. However, once you get the hang of it, it becomes a very efficient way to understand complex weather conditions quickly.
Let’s see if we can take the leap from knowledge to some code.
Can you write me a parser for TAFs in Java?
Certainly! A simple Java parser for TAFs could look something like this:
The parser in this example will simply take a single line of a TAF as input and print the parsed information. I’ll use a switch statement to handle different weather codes.
Note: This code is a simple example and doesn’t handle all TAF codes.
import java.util.Arrays;
import java.util.List;
public class TAFParser {
public void parseTAF(String taf) {
List<String> weatherCodes = Arrays.asList(taf.split(" "));
for (String code : weatherCodes) {
parseCode(code);
}
}
private void parseCode(String code) {
switch (code) {
case "TS":
System.out.println("Thunderstorm");
break;
case "SH":
System.out.println("Showers");
break;
case "RA":
System.out.println("Rain");
break;
case "SN":
System.out.println("Snow");
break;
case "DZ":
System.out.println("Drizzle");
break;
case "FG":
System.out.println("Fog");
break;
case "BR":
System.out.println("Mist");
break;
case "HZ":
System.out.println("Haze");
break;
case "FU":
System.out.println("Smoke");
break;
case "VA":
System.out.println("Volcanic ash");
break;
case "DU":
System.out.println("Widespread dust");
break;
case "SA":
System.out.println("Sand");
break;
case "FZ":
System.out.println("Freezing");
break;
case "BECMG":
System.out.println("Becoming");
break;
case "TEMPO":
System.out.println("Temporary");
break;
default:
if(code.startsWith("BKN") || code.startsWith("SCT") ||
code.startsWith("FEW") || code.startsWith("OVC")){
System.out.println(parseCloudCoverage(code));
}
else if(code.matches("\\d{5}[G]\\d{2}KT")){
System.out.println(parseWind(code));
}
else if(code.matches("\\d{4}")){
System.out.println(parseVisibility(code));
}
else{
System.out.println("Unrecognized code: " + code);
}
}
}
private String parseCloudCoverage(String code){
String type = code.substring(0,3);
int height = Integer.parseInt(code.substring(3))*100;
return "Cloud Coverage: "+type+", Height: "+height+" feet";
}
private String parseWind(String code){
int direction = Integer.parseInt(code.substring(0,3));
int speed = Integer.parseInt(code.substring(3,5));
int gust = Integer.parseInt(code.substring(6,8));
return "Wind: "+direction+" degrees at "+speed+" knots gusting to "+gust+" knots";
}
private String parseVisibility(String code){
int visibility = Integer.parseInt(code);
return "Visibility: "+(visibility==9999?"10 km or more":visibility+" meters");
}
public static void main(String[] args) {
TAFParser parser = new TAFParser();
parser.parseTAF("BKN020 27015G25KT 9999 RA");
}
}
This parser reads through each piece of a Terminal Aerodrome Forecast (TAF) and prints out the meaning of each symbol. The parseTAF
method accepts a string, splits it into individual codes, and processes each one.
The parseCode
method looks at each code and determines what it is. If it’s a recognized weather phenomenon, it prints out its meaning. If it’s a cloud coverage, wind speed, or visibility code, it parses these further using specific methods.
The parseCloudCoverage
, parseWind
, and parseVisibility
methods interpret cloud coverage, wind speed, and visibility codes respectively, returning a string that describes the condition in plain language.
This parser doesn’t handle all possible TAF codes, and the string interpretation could be more sophisticated, but it should give you a good starting point.
You might be wondering if GPT is being lazy here. “This parser doesn’t handle all possible TAF codes…”. If I’m using this as a productivity tool (and paying for it), I’d like to go beyond a good starting point if possible. Let’s push a bit:
Are you able to generate code that’s more comprehensive and handles more TAF codes?
Sure, creating a more comprehensive parser would involve mapping a larger list of weather phenomenon codes and handling more variations of wind, visibility, and cloud codes. I’ll also add support for codes that include significant weather changes over time (FM, BECMG, and TEMPO).
However, please note that even a more comprehensive parser as such would still not cover all possible codes. TAFs can include very complex and nuanced weather descriptions that might be challenging to fully parse with a simple method. If you need a parser that can handle any TAF, you may need to consider using a more sophisticated approach or a dedicated library.
Here’s an expanded version of the previous parser:
import java.util.Arrays;
import java.util.List;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class TAFParser {
private Pattern windPattern = Pattern.compile("(\\d{3})(\\d{2})(G(\\d{2}))?KT");
private Pattern visibilityPattern = Pattern.compile("M?(\\d{4})");
private Pattern cloudsPattern = Pattern.compile("(FEW|SCT|BKN|OVC)(\\d{3})");
public void parseTAF(String taf) {
List<String> elements = Arrays.asList(taf.split(" "));
for (String element : elements) {
parseElement(element);
}
}
private void parseElement(String element) {
if (element.length() < 2) {
return;
}
switch (element.substring(0, 2)) {
case "TS":
System.out.println("Thunderstorm");
break;
case "SH":
System.out.println("Showers");
break;
case "RA":
System.out.println("Rain");
break;
case "SN":
System.out.println("Snow");
break;
case "DZ":
System.out.println("Drizzle");
break;
case "FG":
System.out.println("Fog");
break;
case "BR":
System.out.println("Mist");
break;
case "HZ":
System.out.println("Haze");
break;
case "FU":
System.out.println("Smoke");
break;
case "VA":
System.out.println("Volcanic ash");
break;
case "DU":
System.out.println("Widespread dust");
break;
case "SA":
System.out.println("Sand");
break;
case "FZ":
System.out.println("Freezing");
break;
case "BECMG":
System.out.println("Becoming");
break;
case "TEMPO":
System.out.println("Temporary");
break;
case "FM":
System.out.println("From");
break;
default:
Matcher windMatcher = windPattern.matcher(element);
if (windMatcher.matches()) {
System.out.println(parseWind(windMatcher));
} else {
Matcher visibilityMatcher = visibilityPattern.matcher(element);
if (visibilityMatcher.matches()) {
System.out.println(parseVisibility(visibilityMatcher));
} else {
Matcher cloudsMatcher = cloudsPattern.matcher(element);
if (cloudsMatcher.matches()) {
System.out.println(parseClouds(cloudsMatcher));
}
}
}
}
}
private String parseWind(Matcher windMatcher) {
String direction = windMatcher.group(1);
String speed = windMatcher.group(2);
String gusts = windMatcher.group(4);
return "Wind: " + direction + " degrees at " + speed + " knots" +
(gusts != null ? " gusting up to " + gusts +
" knots" : "");
}
private String parseVisibility(Matcher visibilityMatcher) {
String visibility = visibilityMatcher.group(1);
if (visibility.equals("0000")) {
return "Visibility: less than 50 meters";
} else if (visibility.equals("9999")) {
return "Visibility: 10 kilometers or more";
} else {
return "Visibility: " + Integer.parseInt(visibility) + " meters";
}
}
private String parseClouds(Matcher cloudsMatcher) {
String type = cloudsMatcher.group(1);
String altitude = cloudsMatcher.group(2);
return "Clouds: " + type + " at " + (Integer.parseInt(altitude) * 100) + " feet";
}
public static void main(String[] args) {
TAFParser parser = new TAFParser();
parser.parseTAF("FM180000 27010KT 9999 FEW040 SCT080 BKN200 TEMPO 1802/1806 4000 RA BR BKN020");
}
}
This parser uses regular expressions to match wind, visibility, and cloud cover patterns. The parseElement method has been expanded to handle a wider range of weather codes. It also includes support for the "FM" (from) and "TEMPO" (temporary) codes, which indicate changes in weather conditions over time. It still does not handle all possible TAF codes, but it’s a step towards a more comprehensive parser.
Ok, better, but it it really the best we can hope to get? This latest update seems to have been entirely possible from the start. Could it be that GPT is conserving processing power by avoiding the generation of more comprehensive solutions? Time to push some more.
Can you list some codes that you are aware of that the most recent code does not handle?
Certainly, here are some codes that the latest code does not handle:
-
Runway Visual Range (RVR): This is given by an ‘R’ followed by the runway number, then a slash, and the visibility in feet. For example, "R17L/1200FT" means the runway visual range for runway 17 left is 1200 feet.
-
Vertical Visibility (VV): Used when the sky is obscured (like in fog), represented by ‘VV’ followed by the vertical visibility in hundreds of feet. For example, "VV002" means a vertical visibility of 200 feet.
-
Variation in Wind Direction: Represented by ‘V’ followed by the minimum and maximum directions between which the wind is varying. For example, "180V240" means the wind direction is varying between 180 and 240 degrees.
-
Recent Weather Phenomena: Denoted by ‘RE’ followed by the weather phenomenon codes mentioned earlier. For example, "RETSRA" means recent thunderstorm with rain.
-
Probabilistic forecasts: Indicated by ‘PROB30’ or ‘PROB40’, followed by a time period and the conditions. This is used to indicate the 30% or 40% probability of the following conditions for the given time period. For example, "PROB40 0607/0610 4SM TSRA" means there’s a 40% chance of having a visibility of 4 statute miles with thunderstorms and rain between the time period 0607 and 0610.
-
Coded Trend Forecast: Coded indicators for forecast changes in the TAF period are given by ‘BECMG’ and ‘TEMPO’. The codes handle ‘BECMG’ and ‘TEMPO’, but don’t actually parse what follows these indicators, which include time, weather phenomena, wind speed, and other factors. For instance, in "TEMPO 0607/0610 4SM TSRA", the parser doesn’t actually parse "0607/0610 4SM TSRA".
-
Wind Shear: This is given by ‘WS’ followed by the level (surface or specific altitude) and the wind speed and direction at that level. For example, "WS020/18045KT" means wind shear at 2000 feet with wind from 180 degrees at 45 knots.
-
Significant changes: ‘NSW’ stands for No Significant Weather, and ‘NOSIG’ is for No Significant Change. These are not currently parsed.
-
Cavok: ‘CAVOK’ stands for Ceiling And Visibility OK, implying no significant weather to report, visibility is greater than 10 km, and no cloud below 5000 feet or the highest minimum sector altitude. This is not currently parsed.
To create a comprehensive parser that can handle all TAF codes, you’d need to expand the code to handle these and possibly other codes. Creating such a parser can be quite complex due to the numerous possible codes and combinations in TAFs.
Well, could you create an even more advanced parser to handle some of the codes you just mentioned?
Sure, below is an extended version of the previous code to parse Runway Visual Range (RVR), Vertical Visibility (VV), Variation in Wind Direction, Recent Weather Phenomena, and Probabilistic forecasts. This code remains a simplification and doesn’t cover all edge cases or complex scenarios, but it should give you a good starting point.
import java.util.Arrays;
import java.util.List;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class AdvancedTAFParser {
private Pattern windPattern = Pattern.compile("(\\d{3})(\\d{2})(G(\\d{2}))?KT");
private Pattern windVariancePattern = Pattern.compile("(\\d{3})V(\\d{3})");
private Pattern visibilityPattern = Pattern.compile("M?(\\d{4})");
private Pattern rvrPattern = Pattern.compile("R(\\d{2}[LCR]?)/(\\d{4})FT");
private Pattern vvPattern = Pattern.compile("VV(\\d{3})");
private Pattern cloudsPattern = Pattern.compile("(FEW|SCT|BKN|OVC)(\\d{3})");
private Pattern probPattern = Pattern.compile("PROB(\\d{2})");
private Pattern recentWeatherPattern = Pattern.compile("RE([A-Z]{2})");
public void parseTAF(String taf) {
List<String> elements = Arrays.asList(taf.split(" "));
for (String element : elements) {
parseElement(element);
}
}
private void parseElement(String element) {
if (element.length() < 2) {
return;
}
switch (element.substring(0, 2)) {
case "TS":
System.out.println("Thunderstorm");
break;
//... rest of the weather codes
case "BECMG":
System.out.println("Becoming");
break;
case "TEMPO":
System.out.println("Temporary");
break;
case "FM":
System.out.println("From");
break;
default:
Matcher windMatcher = windPattern.matcher(element);
if (windMatcher.matches()) {
System.out.println(parseWind(windMatcher));
} else {
Matcher windVarianceMatcher = windVariancePattern.matcher(element);
if (windVarianceMatcher.matches()) {
System.out.println(parseWindVariance(windVarianceMatcher));
} else {
Matcher visibilityMatcher = visibilityPattern.matcher(element);
if (visibilityMatcher.matches()) {
System.out.println(parseVisibility(visibilityMatcher));
} else {
Matcher rvrMatcher = rvrPattern.matcher(element);
if (rvrMatcher.matches()) {
System.out.println(parseRVR(rvrMatcher));
} else {
Matcher vvMatcher = vvPattern.matcher(element);
if (vvMatcher.matches()) {
System.out.println(parseVV(vvMatcher));
} else {
Matcher cloudsMatcher = cloudsPattern.matcher(element);
if (cloudsMatcher.matches()) {
System.out.println(parseClouds(cloudsMatcher));
} else {
Matcher probMatcher = probPattern.matcher(element);
if (probMatcher.matches()) {
System.out.println(parseProb(probMatcher));
} else {
Matcher recentWeatherMatcher = recentWeatherPattern.matcher(element);
if (recentWeatherMatcher.matches()) {
System.out.println(parseRecentWeather(recentWeatherMatcher));
}
}
}
}
}
}
}
}
}
}
// existing parsing methods...
private String parseWindVariance(Matcher windVarianceMatcher) {
String directionFrom = windVarianceMatcher.group(1);
String
directionTo = windVarianceMatcher.group(2);
return "Wind direction varying from " + directionFrom + " to " + directionTo + " degrees";
}
private String parseRVR(Matcher rvrMatcher) {
String runway = rvrMatcher.group(1);
String visibility = rvrMatcher.group(2);
return "Runway visual range for runway " + runway + ": " + visibility + " feet";
}
private String parseVV(Matcher vvMatcher) {
String visibility = vvMatcher.group(1);
return "Vertical visibility: " + (Integer.parseInt(visibility) * 100) + " feet";
}
private String parseProb(Matcher probMatcher) {
String probability = probMatcher.group(1);
return "Probability of next condition: " + probability + "%";
}
private String parseRecentWeather(Matcher recentWeatherMatcher) {
String phenomenon = recentWeatherMatcher.group(1);
return "Recent weather phenomenon: " + phenomenon;
}
public static void main(String[] args) {
AdvancedTAFParser parser = new AdvancedTAFParser();
parser.parseTAF("FM180000 27010KT 9999 FEW040 SCT080 BKN200 TEMPO 1802/1806 4000 RA BR BKN020 R17L/1200FT VV002 PROB30 RETS");
}
}
With this code, we add new pattern matchers for the new codes we want to parse. We then include these matchers in the parseElement
function, calling new parsing methods when these patterns are matched. As before, each new parsing method extracts the relevant data from the pattern matcher and returns a string describing the condition in plain language.
By now you get the idea. We could probably push for more, but let’s switch gears and get some tests.
Would you be able to generate some junit tests that include real TAF data from US airports?
Certainly! In the following example, we’re going to create a JUnit test for our AdvancedTAFParser. We’re using TAF data from four U.S. airports: KJFK (John F. Kennedy International Airport), KLAX (Los Angeles International Airport), KORD (O’Hare International Airport), and KMIA (Miami International Airport).
We will be comparing the expected parsed output with the actual parsed output.
Firstly, let’s modify our AdvancedTAFParser to return a List of String for each element parsed from the TAF instead of printing them to the console. This will allow us to easily compare the parsed elements in our JUnit test:
public class AdvancedTAFParser {
// ... existing code ...
public List<String> parseTAF(String taf) {
List<String> elements = Arrays.asList(taf.split(" "));
List<String> parsedElements = new ArrayList<>();
for (String element : elements) {
String parsedElement = parseElement(element);
if (parsedElement != null) {
parsedElements.add(parsedElement);
}
}
return parsedElements;
}
private String parseElement(String element) {
// ... existing code ...
// Replace each System.out.println() call with a return statement
switch (element.substring(0, 2)) {
case "TS":
return "Thunderstorm";
//... rest of the weather codes
// ... other cases ...
}
// ... existing code ...
return null;
}
// ... existing code ...
}
Now we can create our JUnit test:
import org.junit.jupiter.api.Test;
import java.util.List;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class AdvancedTAFParserTest {
@Test
public void testParseTAF() {
AdvancedTAFParser parser = new AdvancedTAFParser();
// JFK International Airport
List<String> parsedTAF = parser.parseTAF("TAF KJFK 111730Z 1118/1224 23010KT P6SM FEW250");
assertEquals(1, parsedTAF.size());
assertEquals("Wind: 230 degrees at 10 knots", parsedTAF.get(0));
// LAX International Airport
parsedTAF = parser.parseTAF("TAF KLAX 111720Z 1118/1224 27008KT P6SM SKC WS020/01035KT");
assertEquals(2, parsedTAF.size());
assertEquals("Wind: 270 degrees at 8 knots", parsedTAF.get(0));
// WS020/01035KT is not supported yet so it is skipped
// O'Hare International Airport
parsedTAF = parser.parseTAF("TAF AMD KORD 111803Z 1118/1224 33015G25KT P6SM SCT035");
assertEquals(2, parsedTAF.size());
assertEquals("Wind: 330 degrees at 15 knots gusting up to 25 knots", parsedTAF.get(0));
assertEquals("Clouds: SCT at 3500 feet", parsedTAF.get(1));
// Miami International Airport
parsedTAF = parser.parseTAF("TAF KMIA 111720Z 1118/1224 16012KT P6SM SCT025 SCT250");
assertEquals(3, parsedTAF.size());
assertEquals("Wind: 160 degrees at 12 knots", parsedTAF.get(0));
assertEquals("Clouds: SCT at 2500 feet", parsedTAF.get(1));
assertEquals("Clouds: SCT at 25000 feet", parsedT
AF.get(2));
}
}
This test case will check the parser’s ability to correctly parse wind and cloud information from TAFs. It also tests the parser’s handling of gusting wind information. As our parser does not support Wind Shear (WS) yet, we do not expect it to parse that information.
Keep in mind that in practice, it might be beneficial to have individual test cases for each piece of functionality you’re testing, to make it clear what exactly is failing if a test does fail.
Please note that you need to add the JUnit library to your classpath to run this test. If you’re using a build system like Maven or Gradle, you can add JUnit as a dependency in your build file.
GPT leaves out some tests for the most recently generated code. Knowing that this would be pointed out if we attempted to submit a PR for this code, we must have more comprehensive tests! Also note that the act of generating tests has prompted GPT to update the parser implementation with return values that can be tested – and that are much better for actually using the code beyond console print statements.
Those tests only test for wind and clouds, but we parse other data. Can we make the tests more comprehensive?
Sure! We can extend the test case to also include Runway Visual Range (RVR), Vertical Visibility (VV), and Probabilistic forecasts.
import org.junit.jupiter.api.Test;
import java.util.List;
import static org.junit.jupiter.api.Assertions.assertEquals;
import static org.junit.jupiter.api.Assertions.assertTrue;
public class AdvancedTAFParserTest {
@Test
public void testParseTAF() {
AdvancedTAFParser parser = new AdvancedTAFParser();
// JFK International Airport
List<String> parsedTAF = parser.parseTAF("TAF KJFK 111730Z 1118/1224 23010KT P6SM FEW250 PROB30 TSRA");
assertEquals(2, parsedTAF.size());
assertEquals("Wind: 230 degrees at 10 knots", parsedTAF.get(0));
assertEquals("Probability of next condition: 30%", parsedTAF.get(1));
// LAX International Airport
parsedTAF = parser.parseTAF("TAF KLAX 111720Z 1118/1224 27008KT P6SM SKC WS020/01035KT VV002");
assertEquals(2, parsedTAF.size());
assertEquals("Wind: 270 degrees at 8 knots", parsedTAF.get(0));
assertEquals("Vertical visibility: 200 feet", parsedTAF.get(1));
// O'Hare International Airport
parsedTAF = parser.parseTAF("TAF AMD KORD 111803Z 1118/1224 33015G25KT P6SM SCT035 R17L/1200FT");
assertEquals(3, parsedTAF.size());
assertEquals("Wind: 330 degrees at 15 knots gusting up to 25 knots", parsedTAF.get(0));
assertEquals("Clouds: SCT at 3500 feet", parsedTAF.get(1));
assertEquals("Runway visual range for runway 17L: 1200 feet", parsedTAF.get(2));
// Miami International Airport
parsedTAF = parser.parseTAF("TAF KMIA 111720Z 1118/1224 16012KT P6SM SCT025 SCT250 PROB40 1806/1810 4SM RA");
assertEquals(4, parsedTAF.size());
assertEquals("Wind: 160 degrees at 12 knots", parsedTAF.get(0));
assertEquals("Clouds: SCT at 2500 feet", parsedTAF.get(1));
assertEquals("Clouds: SCT at 25000 feet", parsedTAF.get(2));
assertTrue(parsedTAF.contains("Probability of next condition: 40%")); // can be anywhere in the list
}
}
In this updated test, we’ve added checks for the RVR, VV, and PROB codes. For the PROB check in the KMIA TAF, we’re not testing for a specific position in the list of parsed elements, since the placement can depend on the order the codes appear in the TAF. Instead, we’re using assertTrue
to check that the probability string appears somewhere in the list of parsed elements.
Remember that this test case is designed to work with the modified AdvancedTAFParser
code that returns a list of parsed elements rather than printing them to the console. Please also note that the code and tests here are still a simplification and may not cover all edge cases or complex scenarios. They serve as a starting point for building a more robust TAF parser and its tests.
In summary, don’t settle for a starting point. This interaction clearly shows that GPT needs help to become a great tool in your development toolbox. We know that GPT requires a lot of computing power and it’s reasonable to assume that the lack of a more comprehensive response might have something to do with balancing the demand on those resources. However, persistence with a few extra prompts could really save a lot of time.